I am a new chef in React world. Just started learning to prepare some tasty recipe what others like. Suddenly I found myself in a situation where I tried to prepare a menu, but it took lots of hard work to prepare the ingredient. I was looking for a tool and got one that made my day easy.
Enough talk let’s look into it.
What is the React recipe?
I have a very tiny application and I would like to pass some data from top level components to some of its child component. Consider the following diagram. For example, I would like to send the logged in to user information from App component to User Info component and Side bar component. How will it do that?
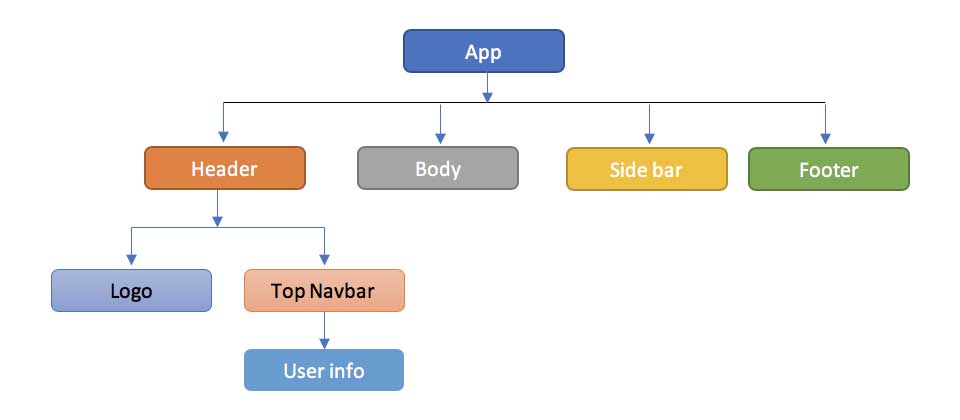
In a typical React application, data is passed top-down (parent to child) via props, but this can be cumbersome if you have many components within an application.The way is to pass the data through all the components. Like below:
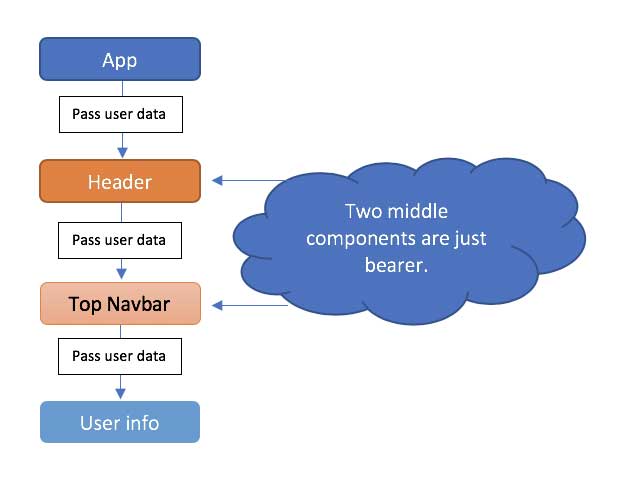
This is not a smart way. We are smart developers.
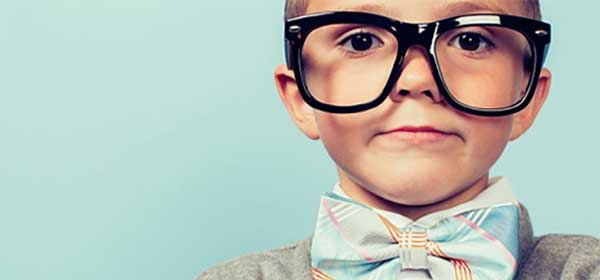
Is there any better approach? Yes, got it. Redux.
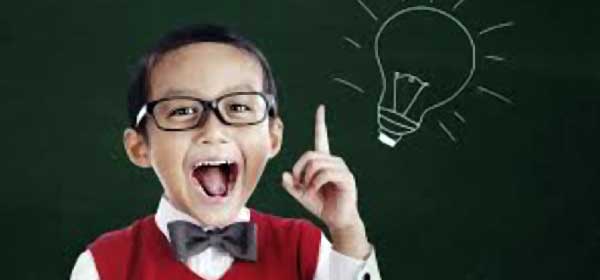
Uh. But Redux is so complex and need to write lots of boilerplate code. What should I do now?
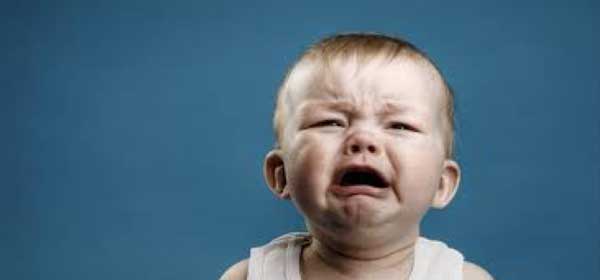
Here is the tool to reduce the hard work. Context.
What is Context?
Context provides a way to share values between components without having to explicitly pass a prop through every level of the tree.
So, if we use context, we can rewrite the diagram as below:
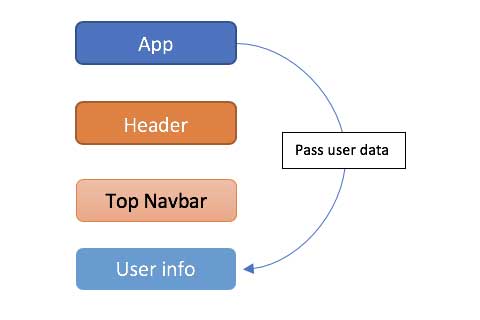
Isn’t that cool? Let’s look at the code now. First let’s create a context.
UserContext.js:
Let’s create a context first. The context has a Provider and Consumer.
import React from 'react';
export const {Provider, Consumer} = React.createContext();
Code language: JavaScript (javascript)
App.js:
Let’s use the Provider here to provide some data for the Consumers. It takes one parameter name value which can accept any object. Here we have set the user object. Keep it mind that when the Provider value changes then the Consumers updated automatically.
import React, { Component } from 'react';
import Header from './components/Header';
import { Provider } from './context/UserContext';
class App extends Component {
state = {
user: {
name: 'Ixora'
}
}
handleClick = () => {
this.setState({
user: {
name: 'Ixora Solution'
}
})
}
render() {
return (
<div>
<h1>App</h1>
<Provider value={this.state.user.name}>
<Header ></Header>
</Provider>
<button onClick={()=> this.handleClick()}>Click</button>
</div>
);
}
}
export default App;
Code language: JavaScript (javascript)
Header.js
import React, { Component } from 'react';
import TopNavbar from './TopNavbar';
class Header extends Component {
render() {
return (
<div>
<h1>Header</h1>
<TopNavbar></TopNavbar>
</div>
);
}
}
export default Header;
Code language: JavaScript (javascript)
TopNavbar.js
import React, { Component } from 'react';
import UserInfo from './UserInfo';
class TopNavbar extends Component {
render() {
return (
<div>
<h1>TopNavbar</h1>
<UserInfo></UserInfo>
</div>
);
}
}
export default TopNavbar;
Code language: JavaScript (javascript)
UserInfo.js:
We have consumed the data which were sent by the Provider.
import React, { Component } from 'react';
import { Consumer } from '../context/UserContext';
class UserInfo extends Component {
render() {
return (
<div>
<h1>UserInfo</h1>
<Consumer>
{(name) => (
<h1>Hello {name}</h1>
)}
</Consumer>
</div>
);
}
}
export default UserInfo;
Code language: JavaScript (javascript)
How do you feel now?
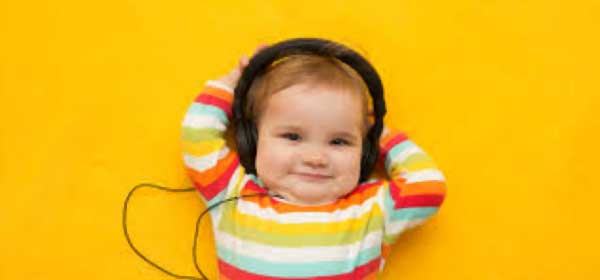
In conclusion, this blog post delved into the topic of context in React and its significance in facilitating communication between components. By leveraging context, developers can efficiently share data throughout their applications without the burden of passing props down the component tree. Whether it’s sharing theme data, authentication state, or any other frequently used information, context proves to be a valuable tool in simplifying data management in React applications. By implementing the concepts and techniques discussed in this blog post, you can enhance your React development skills and create more scalable and maintainable applications.
But there are some caveats of this recipe. Please read more about it at https://reactjs.org/docs/context.html
Hope this will help to understand ReactJS “Context”. You can leave comments below for any help or confusion. I will try to address those as soon possible. Also, you can contact iXora Solution ReactJS Team for any assistance on your project implementation at Contact-iXoraReactJS
Add a Comment